Looking to safeguard your web apps? Here’s a cross site scripting example that demonstrates the havoc XSS attacks can cause when input sanitization is overlooked. Imagine a comment field on your favorite blog—one minute it’s benign user chatter, the next it’s a malicious script stealing session cookies. In the next few minutes, you’ll learn the fundamentals of cross-site scripting, explore a real-world cross-site scripting example, and discover how xss attacks operate. By the end of this guide, you’ll know exactly how to detect, exploit, and—most importantly—prevent these critical web vulnerabilities.
What Is Cross-Site Scripting?
Cross-Site Scripting (XSS) is a client-side code injection attack, where attackers inject malicious scripts into webpages viewed by other users. A classic cross site scripting example involves an attacker submitting a crafted payload in a comment form. When an unsuspecting visitor loads that comment, the script executes in their browser, granting the attacker access to cookies, session tokens, or even the entire DOM.
xss attacks are categorized by where the malicious script is stored or executed:
-
Stored XSS – The payload is permanently stored on the target server (e.g., in a database).
-
Reflected XSS – The payload is embedded in a server response (e.g., in a search result) and reflected back to the user.
-
DOM-based XSS – The vulnerability exists in client-side code, where the payload is processed by JavaScript in the browser.
Why XSS Attacks Matter
-
User data theft: Through a simple cross site scripting example, attackers can hijack user sessions, steal credentials, or log keystrokes.
-
Reputation damage: If your site becomes a vector for xss attacks, visitors lose trust, and compliance requirements may be violated.
-
Regulatory fines: Data breaches from XSS can trigger GDPR or CCPA penalties, potentially costing millions.
📖Related Reading
Types of XSS with Examples
1. Stored XSS
In a classic cross-site scripting example, an attacker submits:
into a comment form. The server saves it to its database and renders it back to every visitor. When loaded, the victim’s browser sends their cookie to the attacker.
2. Reflected XSS
A search form echoes user input:
The server injects the query into the HTML response without sanitization. On page load, the script runs—proof of concept for xss attacks that rely on user-supplied URLs.
3. DOM-Based XSS
In client-side code:
Visiting:
causes the image error handler to fire, exemplifying a DOM payload in action.
Anatomy of an XSS Attack
-
Reconnaissance: Identify user-input fields (forms, URL parameters, headers).
-
Payload crafting: Write a minimal script—often under 100 characters—that exfiltrates data or redirects the user.
-
Injection: Submit the payload via POST, GET, or client-side code manipulation.
-
Execution: Wait for a victim to load the infected page; the payload triggers in their browser.
-
Exfiltration: Data is sent to the attacker’s server, completing the breach.
Potential Impacts
-
Session hijacking: Attackers gain unauthorized access to user accounts.
-
Defacement: Malicious scripts modify page content, or phish credentials.
-
Drive-by downloads: Payloads load secondary malware on victim machines.
-
Keylogging: Keystrokes are captured and sent away, exposing passwords.
How to Detect XSS Vulnerabilities
-
Automated scanners: Tools like OWASP ZAP or Burp Suite can crawl and test inputs with typical XSS payloads.
-
Code review: Look for unsanitized uses of
innerHTML
,document.write
, or server-side echoing functions without escaping. -
Penetration testing: Manual tests—injecting simple scripts into forms, headers, and URLs—often reveal vulnerable spots.
-
Content Security Policy (CSP) reports: Configure CSP with
report-uri
to get notified when inline scripts or eval are used.
Prevention Techniques
1. Input Sanitization & Output Encoding
-
Escape HTML entities (
<
→<
,>
→>
) before rendering user input. -
Use libraries like OWASP Java Encoder or Microsoft AntiXSS.
2. Content Security Policy (CSP)
CSP blocks inline scripts and external sources unless explicit, mitigating many xss attacks.
3. HTTP-Only & Secure Cookies
Mark cookies as HttpOnly
and Secure
so that JavaScript can’t read them:
4. Framework Safeguards
Modern frameworks (React, Angular, Vue) auto-escape interpolated values. Leverage their built-in defenses and avoid bypassing them.
5. Use Trusted Libraries
For rich text editors or user-generated content, deploy sanitization libraries (e.g., DOMPurify) to cleanse HTML.
Cross-Site Scripting Example: A Walkthrough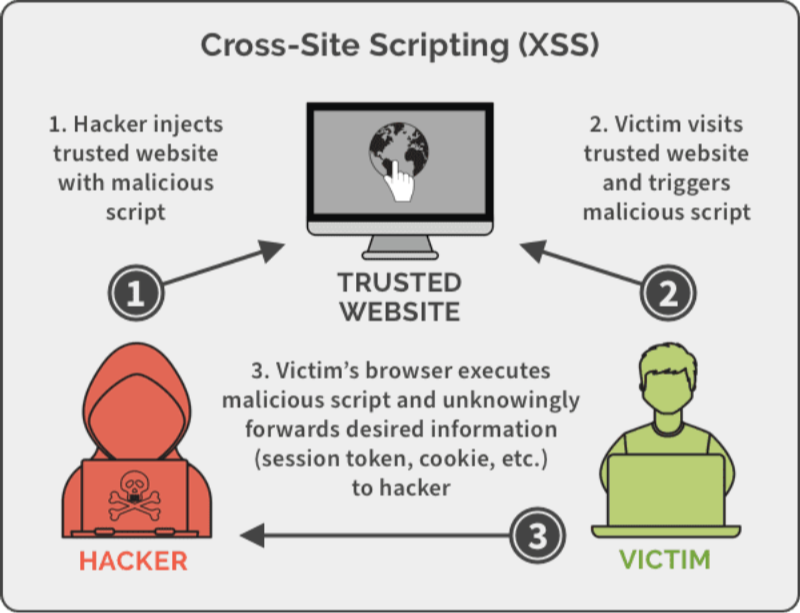
Let’s simulate a stored XSS attack on a rudimentary blog comments section:
-
Vulnerable Code (Server-Side, PHP):
-
Attacker Payload:
-
Result: Every visitor’s browser automatically sends cookies to
attacker.com
, enabling session hijacking.
By studying this cross site scripting example, developers see how missing sanitization on input fields directly leads to account compromise.
How Best free VPN Complements Your XSS Defenses
While sanitizing inputs and enforcing a strict Content Security Policy are your first lines of defense against xss attacks, there’s another tool you can deploy to lock down your browsing and testing environments: UFO VPN. Here’s how it fits into your security toolkit:
-
Encrypted Tunneling for Safe Testing
When you’re probing your own applications for vulnerabilities, UFO VPN’s encrypted tunnel ensures that even if an XSS payload momentarily redirects traffic or exfiltrates data, it stays contained within a secure channel—keeping your production environment and real user data out of harm’s way. -
Geolocation-Based Testing
Some XSS exploits behave differently depending on the user’s region or language settings. UFO VPN lets you switch IP locations instantly, so you can verify that your defenses hold up under every regional configuration and catch edge-case XSS vectors before attackers do. -
Bypassing ISP-Level Filters
Certain ISPs or network firewalls may filter out scripts or alter payloads, skewing your test results. By routing your testing sessions through UFO VPN’s private servers, you get a consistent, unfiltered view—ensuring your manual and automated XSS tests are accurate. -
Privacy and Anonymity During Bug Bounties
If you’re hunting for XSS vulnerabilities on third-party bug-bounty programs, UFO VPN masks your real IP and protects your identity, so you can responsibly disclose findings without exposing your own network footprint.
Combine UFO VPN with automated scanners—run OWASP ZAP or Burp Suite through the VPN, then analyze results knowing every injection and callback is shielded by robust encryption.
Frequently Asked Questions
Q1: What’s the simplest cross-site scripting example?
A classic demo injects <script>alert('XSS')</script>
into a form and observes a popup, showcasing XSS attacks in under five seconds.
Q2: Can HTTPS prevent XSS?
HTTPS encrypts data in transit but does not sanitize malicious input, so it doesn’t stop XSS attacks by itself.
Q3: Are single-page applications immune to XSS?
No. SPAs rely heavily on client-side code; improper handling of user data in JavaScript can still lead to XSS attacks.
Q4: How does CSP help with XSS prevention?
CSP restricts where scripts may be loaded from, blocking inline scripts and untrusted domains, dramatically reducing XSS risk.
Q5: What’s the difference between XSS and SQL injection?
XSS targets client-side code by injecting scripts; SQL injection targets server-side databases by injecting SQL commands.
Conclusion
Through a detailed cross site scripting example and exploration of how xss attacks work, you now have the knowledge to identify, exploit (for testing), and most importantly, prevent XSS in your applications. By combining rigorous input sanitization, strategic Content Security Policies, secure cookie flags, and modern framework best practices, you’ll close the door on these pervasive threats. Implement these safeguards today to keep your users—and your reputation—safe from the next wave of cross-site scripting exploits.